How To Add Gifs in React Native Android and iOS
Published On: 2024-02-12
Posted By: Harish
We can add an image simply with react native's <Image>
tag. Like images, we can show gifs in iOS apps without any external dependencies. But for android, we have to add an extra dependency.
There are many ways to show gifs in react native apps. In this post we will see a commonly used way to show gifs using fresco for android apps.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init GifRN
Add a Gif image
If you want to store gif locally, add a gif image by creating a new folder like assets
(probably outside of src directory) or if you have a gif URL, copy the url.
Import an <Image>
tag and use it to show this animated gif. Get the located gif relative path pass to Image tag source.
//app.tsx
const SunGif = require('../../assets/sun.gif');
return (
...
<Image
source={SunGif} // source={{uri: 'example.com/image.gif'}}
style={styles.image}
/>
...
)
To use an URL, pass it as uri in source, source={{ uri: 'example.com/image.gif'}}
. Now run the project in iOS and android devices or emulators.
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
You will see the gif animation when ran on an iOS emulator or device. But in android, we have an extra dependency to add for animation to work.
For that. open android/app/build.gradle
file and add fresco dependency and fresco's animated dependency.
//android/app/build.gradle
dependencies {
...
...
// The version of react-native is set by the React Native Gradle Plugin
implementation("com.facebook.react:react-android")
implementation("com.facebook.react:flipper-integration")
//Fresco - For Animated Gifs in React Native Android
implementation 'com.facebook.fresco:fresco:3.1.3' <--Add this
implementation 'com.facebook.fresco:animated-gif:3.1.3' <--Add this
...
...
}
Check for the latest fresco dependency version by visiting, Fresco Official Site.
Now clean and build the project in android.
#for Mac
./gradlew clean build
#for Windows
gradle clean build
Now you will see an animated gif in android.
Android
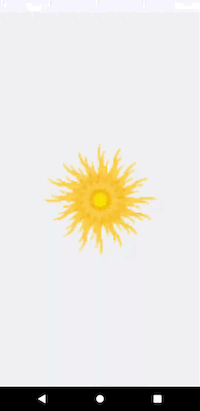
iOS
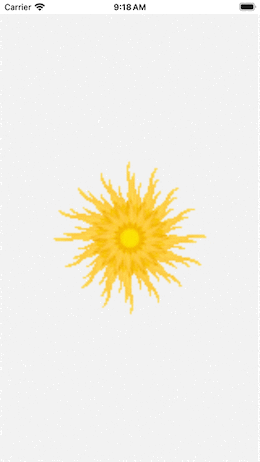
Sun GIF by Peace,love,happiness from Pixabay
Complete code,
//app.tsx
import {
View,
Image,
StyleSheet,
} from "react-native";
const SunGif = require('./assets/sun.gif');
export const App = () => {
return (
<View style={styles.container}>
<Image
source={SunGif}
style={styles.image}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
},
image: {
width: 300,
height: 300,
},
});