Get Dynamic Width and Height of an Image in React Native
Published On: 2024-05-16
Posted By: Harish
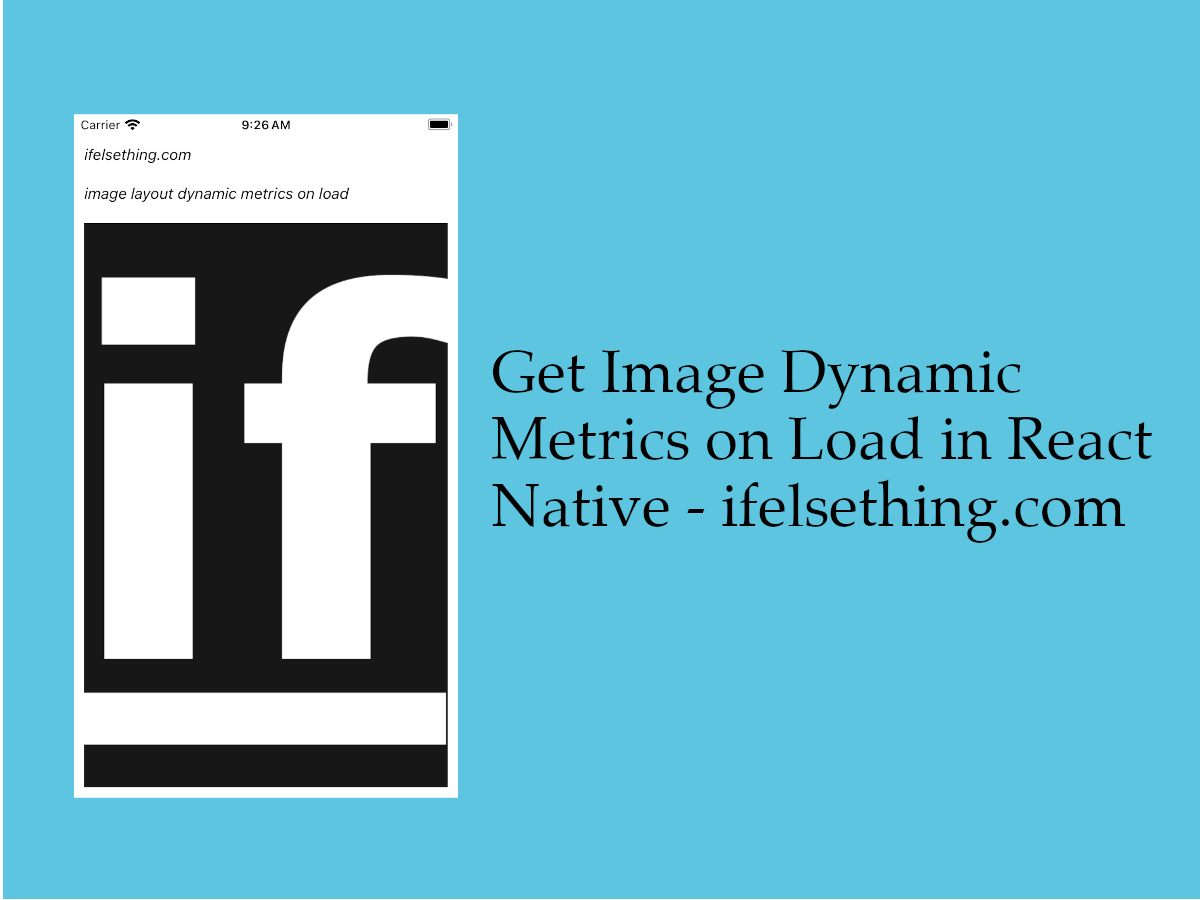
If you want the position of a loaded image or want to get the dynamic width and height of the image, we can use the onLayout callback of the Image component.
Lets see this in action.
Create A New Project
Create a new react-native project by using npx. Check documentation for creating a new react native project.
npx react-native@latest init ImageRN
Example Implementation
We will create a simple image component with a local image file and get its size and position.
Import and add Image
component with a source.
//App.tsx
...
import { Image } from 'react-native';
...
<Image
style={styles.image}
resizeMode="cover"
source={require('./assets/fav.png')}
/>
...
//styles
image: {
flexShrink: 1,
width: '100%'
}
If we run the app,
#for Android
npx react-native run-android
#for ios
npx react-native run-ios
We will see a completely filled large image.
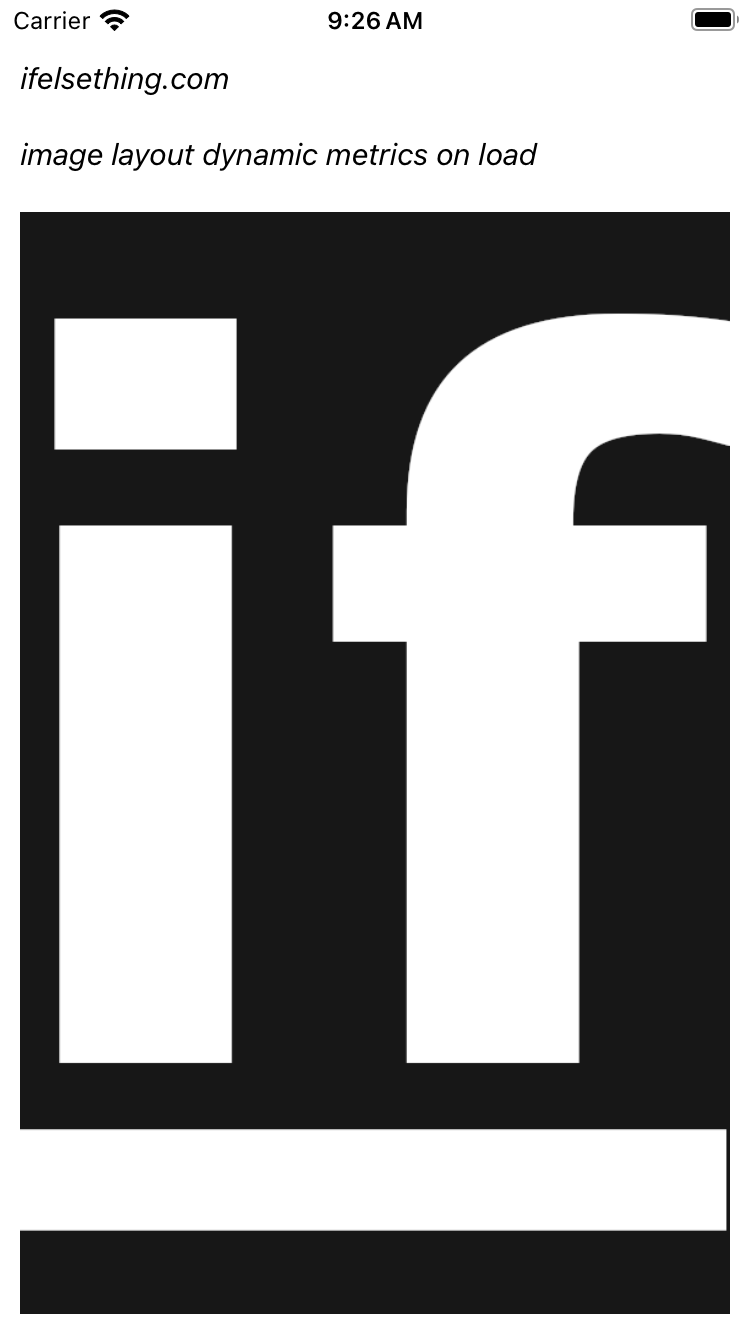
Here we don't know its actual width, height and position.
So, add onLayout
callback to the image component and console log the event's nativeEvent.
...
<Image
...
onLayout={e => console.log(e.nativeEvent)}
/>
...
Now reload the app and after rendering, you will get a similar log like below one.
LOG {"layout": {"height": 551, "width": 355, "x": 0, "y": 76}, "target": 139}
From the above log, we can see its rendered dynamic metrics.
Complete code of our example,
//App.tsx
import React from "react";
import {
Text,
StyleSheet,
SafeAreaView,
StatusBar,
View,
Image,
} from "react-native";
export default function App() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: 'white' }}>
<StatusBar
barStyle="dark-content"
/>
<View style={styles.container}>
<Text style={styles.text}>
ifelsething.com
</Text>
<Text style={styles.text}>
image layout dynamic metrics on load
</Text>
<Image
onLayout={e => console.log(e.nativeEvent)}
style={styles.image}
resizeMode="cover"
source={require('./assets/fav.png')}
/>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
margin: 10,
gap: 20
},
text: {
fontSize: 15,
color: 'black',
fontStyle: 'italic'
},
image: {
flexShrink: 1,
width: '100%'
}
});